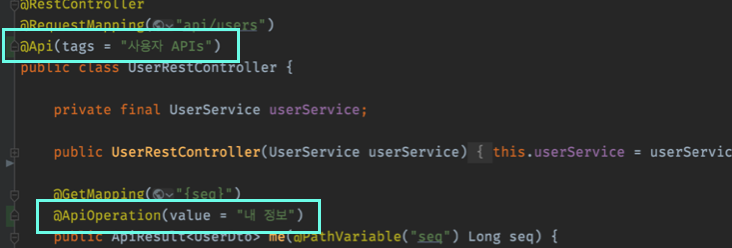
Swagger란?
빌드 시 자동으로 API Spec 문서를 생성해주는 라이브러리
핵심 기능
- API 기능 테스트를 수행할 수 있음
- 소스코드 빌드 시 문서 자동 최신화
의존성 추가
// Spring Boot 2.7.1
implementation "io.springfox:springfox-boot-starter:3.0.0"
Swagger Configuration
@Configuration
public class Swagger2Configure {
// Docket 설정
@Bean
public Docket restApi() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo()) // optional
.securitySchemes(singletonList(apiKey()))
.securityContexts(singletonList(securityContext())) // Security 관련 설정
.produces(singleton("application/json"))
.consumes(singleton("application/json"))
.useDefaultResponseMessages(false)
.select()
.apis(withMethodAnnotation(ApiOperation.class))
.build();
}
private ApiKey apiKey() {
return new ApiKey("apiKey", "내가 지정한 jwt header key name", "header");
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("Test-Server")
.contact(new Contact("username", null, "your-email@address.com"))
.version("1.0.0")
.build();
}
private SecurityContext securityContext() {
return SecurityContext.builder()
.securityReferences(securityReference())
.operationSelector(o -> true)
.build();
}
private List<SecurityReference> securityReference() {
AuthorizationScope authorizationScope = new AuthorizationScope("global", "accessEverything");
AuthorizationScope[] authorizationScopes = new AuthorizationScope[1];
authorizationScopes[0] = authorizationScope;
return singletonList(new SecurityReference("apiKey", authorizationScopes));
}
}
.apiInfo()
Swagger API 문서에 대한 설명 표기
.securitySchemes()
JWT를 읽기 위한 정보
.produces()
응답 객체 반환 시 Response Content-Type 지정
.consumes()
HTTP 요청 시 Request Content-Type 지정
.select()
ApiSelectorBuilder 생성
.apis(withMethodAnnotation(ApiOperation.class))
@ApiOperation이 붙은 항목을 자동으로 문서화한다.
설정이 완료되었다면 어노테이션을 통해 API 명세를 작성한다.
Swagger Annotation
어노테이션 | 설명 |
@Api | Controller 단위로 REST API 메타데이터 명시 |
@ApiOperation | 하나의 REST API 요청 URL에 매핑, 문서화 대상 |
@ApiParam | REST API 호출 시 전달되는 파라미터 설명 |
Controller, Method Spec을 문서화하거나
@PostMapping("exists")
public ApiResult<Boolean> checkEmail(@RequestBody @ApiParam(value= "example: {\"address\": \"test00@gmail.com\"}") HashMap<String, String> request) {
Email email = new Email(request.get("address"));
return OK(userService.findByEmail(email).isPresent());
}
위와 같이 DTO 를 사용하지 않는 HashMap의 명세를 적을 때 사용할 수 있다.
swagger 사용법
셋팅이 완료되었다면 서버를 실행하고
브라우저 주소창에 http://localhost:port번호/swagger-ui/ 을 입력하여 아래와 같은 화면에 접속한다.
사용자 정보로 로그인하는 테스트를 해보자.
Try it out 버튼을 누르면 아래와 같이 text area가 활성화된다.
테스트 데이터 정보를 적고 Execute 버튼을 눌러 실행해보자.
로그인 시 컨트롤러에서 생성 된 JWT 토큰 정보를 반환하게 구현해두면
아래와 같이 Token 정보가 response 된 것을 확인할 수 있다.
토큰을 발급받았으니 이제 인증이 필요한 컨트롤러도 테스트를 할 수 있다.
Authorize 버튼을 클릭해서 발급 된 토큰 값을 입력한다.
토큰 값 입력 후에는 인증 정보가 필요한 "내 정보" Method 에 접근이 가능하다.
'Spring Boot' 카테고리의 다른 글
Spring Security 인증 처리 (2) Filter, AuthenticationManager, AuthenticationProvider, WebSecurityConfigure (0) | 2022.07.26 |
---|---|
Spring Security 인증 처리 (1) Authentication, SecurityContextHolder (0) | 2022.07.23 |
[Spring Boot] JUnit5 로 테스트 하기 (0) | 2022.05.25 |
JWT 를 간단히 정리해보자 (1) | 2022.05.17 |
[Spring Boot] H2 DataBase (0) | 2022.02.14 |